A list of top frequently asked ASP.NET interview questions and answers are given below.
1) What is ASP?
ASP stands for Active Server Pages. It is also known as classic ASP. It is a server-side technology provided by Microsoft which is used to create dynamic and user-friendly web pages. It uses different scripting languages to create dynamic web pages which can be run on any browsers.
1. ASP.NET provides services to allow the creation, deployment, and execution of Web Applications and Web Services.
2. Like ASP, ASP.NET is a server-side technology.
3. Web Applications are built using Web Forms. ASP.NET comes with built-in Web Forms controls, which are responsible for generating the user interface. They mirror typical HTML widgets like text boxes or buttons. If these controls do not fit your needs, you are free to create your own user controls.
4. Web Forms are designed to make building web-based applications as easy as building Visual Basic applications.
4) What is IIS?
IIS stands for Internet Information Services. It is created by Microsoft to provide Internet-based services to ASP.NET Web applications.
5) What is the usage of IIS?
Following are the main usage of IIS:
6) What is a multilingual website?
If a website provides content in many languages, it is known as a multilingual website. It contains multiple copies of its content and other resources, such as date and time, in different languages.
9) What are the advantages of ASP.NET?
ASP.Net is the next generation of ASP technology platform. It is superior to ASP in the following ways:
10) What is the concept of Postback in ASP.NET?
Postback is a request which is sent from a client to the server from the same page user is working with. There is an HTTP POST request mechanism in ASP.NET. It posts a complete page back to the server to refresh the whole page.
If we create a web Page, which consists of one or more Web Controls that are configured to use AutoPostBack (Every Web controls will have their own AutoPostBack property), the ASP.NET adds a special JavaScipt function to the rendered HTML Page. This function is named _doPostBack() . When Called, it triggers a PostBack, sending data back to the web Server.
ASP.NET also adds two additional hidden input fields that are used to pass information back to the server. This information consists of ID of the Control that raised the event and any additional information if needed. These fields will empty initially as shown below,
The following actions will be taken place when a user changes a control that has the AutoPostBack property set to true:
1. On the client side, the JavaScript _doPostBack function is invoked, and the page is resubmitted to the server.
2. ASP.NET re-creates the Page object using the .aspx file.
3. ASP.NET retrieves state information from the hidden view state field and updates the controls accordingly.
4. The Page.Load event is fired.
5. The appropriate change event is fired for the control. (If more than one control has been changed, the order of change events is undetermined.)
6. The Page.PreRender event fires, and the page is rendered (transformed from a set of objects to an HTML page).
7. Finally, the Page.Unload event is fired.
8. The new page is sent to the client.
11) What is the used of "isPostBack" property?
The "IsPostBack" property of page object is used to check that the page is posted back or not.
13) What is the parent class of all web server control?
System.Web.UI.Control class
14) What is the difference between ASP.NET Webforms and ASP.NET MVC?
ASP.NET Webforms uses the page controller approach for rendering layout. In this approach, every page has its controller.
On the other hand, ASP.NET MVC uses the Front Controller approach. In this approach, there is a common controller for all pages.
15) What is the difference between the GET method () and POST method ()?
get method( )
post method( )
On the other hand, the application object is used to store the information and access variables from any page in the application.
17) What is the difference between trace and debug?
Debug class is used to debug builds. Trace class is used for both debug and release builds.
Tracing helps to see the information of issues at the runtime of the application. By default Tracing is disabled.
Tracing has the following important features:
1. We can see the execution path of the page and application using the debug statement.
2. We can access and manipulate trace messages programmatically.
3. We can see the most recent tracing of the data.
Tracing can be done with the following 2 types.
19) What is the difference between file-based dependency and key-based dependency?
File-based dependency: File-based dependency facilitates you to save the dependency on a file in a disk.
Key-based dependency: In key-based dependency, you depend on another cached item.
20) What is the difference between globalization and localization?
Globalization: Globalization is a technique to identify the part of a Web application that is different for different languages and separate it out from the web application.
Localization: In localization, you try to configure a Web application so that it can be supported for a specific language or locale.
21) What is the difference between a page theme and a global theme?
Page Theme: The page theme is applied to particular web pages of the project. It is stored inside a subfolder of the App_Themes folder.
22) What is the difference between early binding and late binding?
Early Binding: In early binding, a non-virtual method is called which is decided at a compile time.
Late Binding: In late binding, a virtual method is called which is decided at runtime.
24) How to sign out from forms authentication?
FormsAuthentication.Signout() method is used to sign out from forms authentication.
25) How to display validation messages in one control?
By the help of ValidationSummary control, we can display all validation messages in one control.
26) What is the difference between authentication and authorization?
Authentication is a process of identifying user whereas authorization is used to check the access rights of an identified user.
27) Which object encapsulates state or data of a user?
Session object.
29) What is ViewState information stored?
It is stored in HTML hidden field.
30) What are the differences between the Response.Write() and Response.Output.Write()?
Response.Write() is used for normal output whereas Response.Output.Write() is used for formatted output.
31) Define the types of configuration files.
There are two types of configuration files:
Application Level config = Web.config.
Machine Level config = Machine.config.
32) What is the difference between Web config and Machine config files?
Web config file is specific to web application whereas Machine config file is specific to machine or server.
There can be multiple web config files in an application but only one machine config file.
33) What is MVC?
MVC stands for Model View Controller. It is a design pattern that is used to separate business logic and presentation logic. It is used to develop the highly customized application.
The Model represents data, View represents presentation and controller acts as an interface between Model and View.
34) What are the built-in objects in ASP.NET?
The major built-in objects are given below:
Application
Session
Context
Request
Response
Server
Trace
35) What do you mean by Role-based security?
Role-based security is used in almost all organization, and the Role-based security assigns certain privileges to each role.
36) What is a cookie?
A Cookie is a small piece of information which is stored at the client side. There are two types of cookie:
Session/Temporary Cookie: valid for a single session
Persistent Cookie: valid for multiple session
Cookies are a State Management Technique that can store the values of control after a post-back. Cookies can store user-specific Information on the client's machine like when the user last visited your site. Cookies are also known by many names, such as HTTP Cookies, Browser Cookies, Web Cookies, Session Cookies and so on. Basically cookies are a small text file sent by the web server and saved by the Web Browser on the client's machine.
List of properties containing the HttpCookies Class:
Domain: Using these properties we can set the domain of the cookie.
Expires: This property sets the Expiration time of the cookies.
HasKeys: If the cookies have a subkey then it returns True.
Name: Contains the name of the Key.
Path: Contains the Virtual Path to be submitted with the Cookies.
Secured: If the cookies are to be passed in a secure connection then it only returns True.
Value: Contains the value of the cookies.
Limitation of the Cookies
The size of cookies is limited to 4096 bytes.
A total of 20 cookies can be used in a single website.
37) What is the default timeout for a cookie?
30 minutes.
38) How would you turn off cookies on a page of a website?
You have to follow the procedures given below:
Use the "Cookie.Discard" property.
It gets or sets the discard flag set by the server.
When set to true, this property instructs the client application not to save the Cookie on the hard disk of the user at the end of the session.
39) Which protocol is used to call web service?
HTTP protocol.
40) What is the file extension of web service?
The File extension of web service is .asmx.
42) What is the use of Global.asax file?
The Global.asax file is used to execute the application-level events and sets application-level variables.
43) What is event bubbling?
When child control sends events to parent, it is termed as event bubbling. Server controls like Data Grid, Data List, and Repeater can have other child controls inside them.
44) What are the different validators in ASP.NET?
ASP.NET validation controls define an important role in validating the user input data. Whenever the user gives input, it must always be validated before sending it across the various layers of an application. There are two types of validation in ASP.NET:
Client-Side Validation
Server-Side Validation
Client-Side Validation: When validation is done on the client browser, it is known as Client-Side Validation. You can use JavaScript to do the Client-Side Validation.
RequiredFieldValidator Control
CompareValidator Control
RangeValidator Control
RegularExpressionValidator Control
CustomFieldValidator Control
ValidationSummary
45) What is the difference between Server.Transfer and Response.Redirect?
In Server.Transfer page processing transfers from one page to the other page without making a round-trip back to the client's browser. This provides a faster response with a little less overhead on the server. The clients url history list or current url Server does not update in case of Server.Transfer.
Response.Redirect is used to redirect the user's browser to another page or site. It performs trip back to the client where the client's browser is redirected to the new page. The user's browser history list is updated to reflect the new address.
46) In which event of page cycle is the ViewState available?
After the Init() and before the Page_Load().
47) What are the different Session state management options available in ASP.NET?
In-Process
Out-of-Process.
In-Process stores the session in memory on the web server.
Out-of-Process Session state management stores data in an external server. The external server may be either a SQL Server or a State Server. All objects stored in session are required to be serializable for Out-of-Process state management.
48) How you can add an event handler?
Using the Attributes property of server side control.
btnSubmit.Attributes.Add("onMouseOver","JavascriptCode();")
49) What are the different types of caching?ASP.NET has 3 kinds of caching :
Output Caching,
Fragment Caching,
Data Caching.
50) List the events in page life cycle.
1) Page_PreInit
2) Page_Init
3) Page_InitComplete
4) Page_PreLoad
5) Page_Load
6) Page_LoadComplete
7) Page_PreRender
8) Render
ASP.NET goes through a series of stages in the life cycle of each page.
Page Start. The Request and Response objects are created.
Page Initialization. All page controls are initialized, and any themes are applied.
Page Load. ASP.NET uses the view state and control state properties to set the control properties. Default values are set in the controls.
Postback event handling. This event is triggered if the same page is loaded again.
Rendering. ASP.NET saves the view state for the page and writes the output of rendering to the output stream. It happens just before the complete web page is sent to the user.
Unload. The rendered page gets sent to the client. ASP.NET unloads page properties and performs cleanup. All unwanted objects are removed from memory.
ASP.NET Page Life Cycle Events
Following are the page life cycle events:
PreInit
PreInit is the first event in page life cycle. It checks the IsPostBack property and determines whether the page is a postback. It sets the themes and master pages, creates dynamic controls and gets and sets profile property values. This event can be handled by overloading the OnPreInit method or creating a Page_PreInit handler.
Init
Init event initializes the control property and the control tree is built. This event can be handled by overloading the OnInit method or creating a Page_Init handler.
InitComplete
InitComplete event allows tracking of view state. All the controls turn on view-state tracking.
LoadViewState
LoadViewState event allows loading view state information into the controls.
LoadPostData
During this phase, the contents of all the input fields defined with the
51) What is Cross Page Posting?
When we click submit button on a web page, the page post the data to the same page. The technique in which we post the data to different pages is called Cross Page posting. This can be achieved by setting POSTBACKURL property of the button that causes the postback. Findcontrol method of PreviousPage can be used to get the posted values on the page to which the page has been posted.
ASP.NET 1.1 provides for web forms posting back only to themselves. In many situations, the solution requires posting to a different web page. The traditional workaround alternatives were to use Response.Redirect and/or Server.Transfer to move to a different page and simulate cross page post-back behavior.
ASP.NET 2.0 provides a feature known as Cross Page PostBack for a web form to post-back to a different web form (other than itself)
How to post to a different page
To set a web form to post back to a different web form, in the source web form, set the PostBackURL property of a control that implements IButtonControl (eg. Button, ImageButton, LinkButton) to the target web form. When the user clicks on this button control, the web form is cross-posted to the target web form. No other settings or code is required in the source web form.
Access source page info within the posted page: FindControl Method
The target web form resulting from the cross-page postback provides a non-null PreviousPage property. This property represents the source page and provides reference to the source web form and its controls.
The controls on the source page can be accessed via the FindControl method on the object returned by the PreviousPage property of the target page.
protected void Page_Load(object sender, EventArgs e)
{
...
TextBox txtStartDate = (TextBox) PreviousPage.FindControl("txtStartDate ");
...
}
At this point the target page does not have any knowledge of the source page. The PreviousPage property is of the type Page. For accessing controls using FindControl, the developer has to presume a certain structure in the source web form. This approach using FindControl has a few limitations. FindControl is dependent on the developer to provide the ids of the controls to access. The code will stop working if the control id is changed in the source web form. The FindControl method can retrieve controls only within the current container. If you need to access a control within another control, you need to first get a reference to the parent control.
Access source page info within the posted page: @PreviousPageType Directive
There is another more direct option to get access to the source page controls if the source page is pre-determined. The @PreviousPageType directive can be used in the target page to strongly type the source page. The directive specifies the source page using either the VirtualPath attribute or the TypeName attribute. The PreviousPage property then returns a strongly typed reference to the source page. It allows access to the public properties of the source page.
SourcePage.aspx:
TargetPage.aspx
<%@ PreviousPageType VirtualPath="sourcepage.aspx" %>
string strFirstName;
strFirstName = PreviousPage.FirstName //Strongly Typed PreviousPage allows direct access to the public properties of the source page.
56) What is boxing and unboxing?
Boxing is assigning a value type to reference type variable.
Unboxing is reverse of boxing ie. Assigning reference type variable to value type variable.
57) What are the components of ADO.NET?
The components of ADO.Net are Dataset, Data Reader, Data Adaptor, Command, connection.
58) What is the difference between ExecuteScalar and ExecuteNonQuery?
ExecuteScalar returns output value where as ExecuteNonQuery does not return any value but the number of rows affected by the query. ExecuteScalar used for fetching a single value and ExecuteNonQuery used to execute Insert and Update statements.
59) Which data type does the RangeValidator control support?
The data types supported by the RangeValidator control are Integer, Double, String, Currency, and Date.
60) What is the difference between an HtmlInputCheckBox control and an HtmlInputRadioButton control?
In HtmlInputCheckBoxcontrol, multiple item selection is possible whereas in HtmlInputRadioButton controls, we can select only single item from the group of items.
61) Which namespaces are necessary to create a localized application?
System.Globalization
System.Resources
63) List all templates of the Repeater control.
ItemTemplate
AlternatingltemTemplate
SeparatorTemplate
HeaderTemplate
FooterTemplate
64) In which event are the controls fully loaded?
Page load event.
65) What is boxing and unboxing?
Boxing is assigning a value type to reference type variable.
Unboxing is reverse of boxing ie. Assigning reference type variable to value type variable.
66) Differentiate strong typing and weak typing
In strong typing, the data types of variable are checked at compile time. On the other hand, in case of weak typing the variable data types are checked at runtime. In case of strong typing, there is no chance of compilation error. Scripts use weak typing and hence issues arises at runtime.
67) How we can force all the validation controls to run?
The Page.Validate() method is used to force all the validation controls to run and to perform validation.
70) What is ASP.NET AJAX?
Ajax stands for Asynchronous JavaScript and XML; in other words Ajax is the combination of various technologies such as a JavaScript, CSS, XHTML, DOM, etc.
AJAX allows web pages to be updated asynchronously by exchanging small amounts of data with the server behind the scenes. This means that it is possible to update parts of a web page, without reloading the entire page.
We can also define Ajax is a combination of client side technologies that provides asynchronous communication between the user interface and the web server so that partial page rendering occurs instead of complete page post back.
Ajax is platform-independent; in other words AJAX is a cross-platform technology that can be used on any Operating System since it is based on XML & JavaScript. It also supports open source implementation of other technology. It partially renders the page to the server instead of complete page post back. We use AJAX for developing faster, better and more interactive web applications.
AJAX uses a HTTP request between web server & browser.
With AJAX, when a user clicks a button, you can use JavaScript and DHTML to immediately update the UI, and spawn an asynchronous request to the server to fetch results.
When the response is generated, you can then use JavaScript and CSS to update your UI accordingly without refreshing the entire page. While this is happening, the form on the users screen doesn't flash, blink, disappear, or stall.
The power of AJAX lies in its ability to communicate with the server asynchronously, using a XMLHttpRequest object without requiring a browser refresh.
Ajax essentially puts JavaScript technology and the XMLHttpRequest object between your Web form and the server.
This is Second Content Place Holder (Default).
Footer...
1) What is ASP?
ASP stands for Active Server Pages. It is also known as classic ASP. It is a server-side technology provided by Microsoft which is used to create dynamic and user-friendly web pages. It uses different scripting languages to create dynamic web pages which can be run on any browsers.
2) What is ASP.NET?
ASP.Net is a specification by Microsoft which is used to create web applications and web services. It is a part of ".Net framework". You can create ASP.Net applications in most of the .Net compatible languages like Visual Basic, C#, etc. ASP.Net provides much better performance than scripting languages.
Advantages of ASP.NET
ASP.Net is a specification by Microsoft which is used to create web applications and web services. It is a part of ".Net framework". You can create ASP.Net applications in most of the .Net compatible languages like Visual Basic, C#, etc. ASP.Net provides much better performance than scripting languages.
Advantages of ASP.NET
Separation of Code from HTML:
To make a clean sweep, with ASP.NET you have the ability to completely separate layout and business logic. This makes it much easier for teams of programmers and designers to collaborate efficiently.
Support for compiled languages:
Developer can use VB.NET and access features such as strong typing and object-oriented programming. Using compiled languages also means that ASP.NET pages do not suffer the performance penalties associated with interpreted code. ASP.NET pages are precompiled to byte-code and Just In Time (JIT) compiled when first requested. Subsequent requests are directed to the fully compiled code, which is cached until the source changes.
Use services provided by the .NET Framework:
The .NET Framework provides class libraries that can be used by your application. Some of the key classes help you with input/output, access to operating system services, data access, or even debugging. We will go into more detail on some of them in this module.
Graphical Development Environment:
Visual Studio .NET provides a very rich development environment for web developers. You can drag and drop controls and set properties the way you do in Visual Basic 6. And you have full IntelliSense support, not only for your code, but also for HTML and XML
To make a clean sweep, with ASP.NET you have the ability to completely separate layout and business logic. This makes it much easier for teams of programmers and designers to collaborate efficiently.
Support for compiled languages:
Developer can use VB.NET and access features such as strong typing and object-oriented programming. Using compiled languages also means that ASP.NET pages do not suffer the performance penalties associated with interpreted code. ASP.NET pages are precompiled to byte-code and Just In Time (JIT) compiled when first requested. Subsequent requests are directed to the fully compiled code, which is cached until the source changes.
Use services provided by the .NET Framework:
The .NET Framework provides class libraries that can be used by your application. Some of the key classes help you with input/output, access to operating system services, data access, or even debugging. We will go into more detail on some of them in this module.
Graphical Development Environment:
Visual Studio .NET provides a very rich development environment for web developers. You can drag and drop controls and set properties the way you do in Visual Basic 6. And you have full IntelliSense support, not only for your code, but also for HTML and XML
State management:
To refer to the problems mentioned before, ASP.NET provides solutions for session and application state management. State information can, for example, be kept in memory or stored in a database. It can be shared across web farms, and state information can be recovered, even if the server fails or the connection breaks down.
Update files while the server is running:
Components of your application can be updated while the server is online and clients are connected. The framework will use the new files as soon as they are copied to the application. Removed or old files that are still in use are kept in memory until the clients have finished.
XML-Based Configuration Files:
Configuration settings in ASP.NET are stored in XML files that you can easily read and edit. You can also easily copy these to another server, along with the other files that comprise your application.
3) What is the difference between the ASP and ASP.NET?
The main difference between ASP and ASP.Net is that ASP is interpreted, while ASP.Net is compiled. ASP uses VBScript, therefore when the ASP page is executed, it is interpreted. On the other hand, ASP.Net uses .Net languages like C# and VB.NET, which is compiled to Microsoft intermediate language.
Here are some points that give the quick overview of ASP.NET.
To refer to the problems mentioned before, ASP.NET provides solutions for session and application state management. State information can, for example, be kept in memory or stored in a database. It can be shared across web farms, and state information can be recovered, even if the server fails or the connection breaks down.
Update files while the server is running:
Components of your application can be updated while the server is online and clients are connected. The framework will use the new files as soon as they are copied to the application. Removed or old files that are still in use are kept in memory until the clients have finished.
XML-Based Configuration Files:
Configuration settings in ASP.NET are stored in XML files that you can easily read and edit. You can also easily copy these to another server, along with the other files that comprise your application.
3) What is the difference between the ASP and ASP.NET?
The main difference between ASP and ASP.Net is that ASP is interpreted, while ASP.Net is compiled. ASP uses VBScript, therefore when the ASP page is executed, it is interpreted. On the other hand, ASP.Net uses .Net languages like C# and VB.NET, which is compiled to Microsoft intermediate language.
Here are some points that give the quick overview of ASP.NET.
1. ASP.NET provides services to allow the creation, deployment, and execution of Web Applications and Web Services.
2. Like ASP, ASP.NET is a server-side technology.
3. Web Applications are built using Web Forms. ASP.NET comes with built-in Web Forms controls, which are responsible for generating the user interface. They mirror typical HTML widgets like text boxes or buttons. If these controls do not fit your needs, you are free to create your own user controls.
4. Web Forms are designed to make building web-based applications as easy as building Visual Basic applications.
4) What is IIS?
IIS stands for Internet Information Services. It is created by Microsoft to provide Internet-based services to ASP.NET Web applications.
5) What is the usage of IIS?
Following are the main usage of IIS:
IIS is used to make your computer to work as a Web server and provides the functionality to develop and deploy Web applications on the server.
IIS handles the request and response cycle on the Web server.
IIS also offers the services of SMTP and FrontPage server extensions.
The SMTP is used to send emails and use FrontPage server extensions to get the dynamic features of
IIS, such as form handler.
6) What is a multilingual website?
If a website provides content in many languages, it is known as a multilingual website. It contains multiple copies of its content and other resources, such as date and time, in different languages.
7) What is caching? Explain.
Caching is the technique which facilitates you to store frequently used items in memory so that they can be accessed more quickly.
Caching is the technique which facilitates you to store frequently used items in memory so that they can be accessed more quickly.
8) what are the main requirements for caching?
By caching the response, your request is served by the response already stored in memory.
By caching the response, your request is served by the response already stored in memory.
You must be very careful while choosing the items to cache because Caching incurs overhead.
A frequently used web form which data doesn't frequently change is good for caching.
A cached web form freezes form?s server-side content, and changes to that content do not appear until the cache is refreshed.
A frequently used web form which data doesn't frequently change is good for caching.
A cached web form freezes form?s server-side content, and changes to that content do not appear until the cache is refreshed.
9) What are the advantages of ASP.NET?
ASP.Net is the next generation of ASP technology platform. It is superior to ASP in the following ways:
Highly Scalable
Compiled Code
User Authentication
Language Support
Third party control
Configuration and Deployment are easy.
Object and Page caching
Strict coding requirements
Compiled Code
User Authentication
Language Support
Third party control
Configuration and Deployment are easy.
Object and Page caching
Strict coding requirements
10) What is the concept of Postback in ASP.NET?
Postback is a request which is sent from a client to the server from the same page user is working with. There is an HTTP POST request mechanism in ASP.NET. It posts a complete page back to the server to refresh the whole page.
If we create a web Page, which consists of one or more Web Controls that are configured to use AutoPostBack (Every Web controls will have their own AutoPostBack property), the ASP.NET adds a special JavaScipt function to the rendered HTML Page. This function is named _doPostBack() . When Called, it triggers a PostBack, sending data back to the web Server.
ASP.NET also adds two additional hidden input fields that are used to pass information back to the server. This information consists of ID of the Control that raised the event and any additional information if needed. These fields will empty initially as shown below,
The following actions will be taken place when a user changes a control that has the AutoPostBack property set to true:
1. On the client side, the JavaScript _doPostBack function is invoked, and the page is resubmitted to the server.
2. ASP.NET re-creates the Page object using the .aspx file.
3. ASP.NET retrieves state information from the hidden view state field and updates the controls accordingly.
4. The Page.Load event is fired.
5. The appropriate change event is fired for the control. (If more than one control has been changed, the order of change events is undetermined.)
6. The Page.PreRender event fires, and the page is rendered (transformed from a set of objects to an HTML page).
7. Finally, the Page.Unload event is fired.
8. The new page is sent to the client.
11) What is the used of "isPostBack" property?
The "IsPostBack" property of page object is used to check that the page is posted back or not.
12) How do you identify that the page is PostBack?
There is a property named "IsPostBack" property in Post object, which can be checked to know that the page is posted back.
There is a property named "IsPostBack" property in Post object, which can be checked to know that the page is posted back.
13) What is the parent class of all web server control?
System.Web.UI.Control class
14) What is the difference between ASP.NET Webforms and ASP.NET MVC?
ASP.NET Webforms uses the page controller approach for rendering layout. In this approach, every page has its controller.
On the other hand, ASP.NET MVC uses the Front Controller approach. In this approach, there is a common controller for all pages.
15) What is the difference between the GET method () and POST method ()?
get method( )
post method( )
1. Get method Data is affixed to the URL.
Post method Data is not affixed to the URL.
2. Get method Data is not secured.
Post method Data is secured.
3. Get method Data transmission is faster in this method.
Post method Data transmission is comparatively slow.
4. Get method It is a single call system.
Post method is a two call system.
5. Get method Only a limited amount of data can be sent.
Post method - A large amount of data can be sent.
6. Get method It is a default method for many browsers.
Post method is not set as default. It should be explicitly specified.
16) What is the difference between session object and application object?
The session object is used to maintain the session of each user. A session id is generated if a user enters in the application and when the user leaves the application, the session id is automatically deleted.
Post method Data is not affixed to the URL.
2. Get method Data is not secured.
Post method Data is secured.
3. Get method Data transmission is faster in this method.
Post method Data transmission is comparatively slow.
4. Get method It is a single call system.
Post method is a two call system.
5. Get method Only a limited amount of data can be sent.
Post method - A large amount of data can be sent.
6. Get method It is a default method for many browsers.
Post method is not set as default. It should be explicitly specified.
16) What is the difference between session object and application object?
The session object is used to maintain the session of each user. A session id is generated if a user enters in the application and when the user leaves the application, the session id is automatically deleted.
On the other hand, the application object is used to store the information and access variables from any page in the application.
17) What is the difference between trace and debug?
Debug class is used to debug builds. Trace class is used for both debug and release builds.
Tracing helps to see the information of issues at the runtime of the application. By default Tracing is disabled.
Tracing has the following important features:
1. We can see the execution path of the page and application using the debug statement.
2. We can access and manipulate trace messages programmatically.
3. We can see the most recent tracing of the data.
Tracing can be done with the following 2 types.
1. Page Level: When the trace output is displayed on the page and for the page-level tracing we need to set the property of tracing at the page level.
<%@ Page Trace="true" Language="C#"
2. Application: Level: In Application-Level tracing the information is stored for each request of the application. The default number of requests to store is 10. But if you want to increase the number of requests and discard the older request and display a recent request then you need to set the property in the web.config file.
<%@ Page Trace="true" Language="C#"
2. Application: Level: In Application-Level tracing the information is stored for each request of the application. The default number of requests to store is 10. But if you want to increase the number of requests and discard the older request and display a recent request then you need to set the property in the web.config file.
18) What is the difference between client-side and server-side validations in WebPages?
The client-side validation happens at the client's side with the help of JavaScript and VBScript. This validation has occurred before the Web page is sent to the server.
The server-side validation happens at the server side.
The client-side validation happens at the client's side with the help of JavaScript and VBScript. This validation has occurred before the Web page is sent to the server.
The server-side validation happens at the server side.
19) What is the difference between file-based dependency and key-based dependency?
File-based dependency: File-based dependency facilitates you to save the dependency on a file in a disk.
Key-based dependency: In key-based dependency, you depend on another cached item.
20) What is the difference between globalization and localization?
Globalization: Globalization is a technique to identify the part of a Web application that is different for different languages and separate it out from the web application.
Localization: In localization, you try to configure a Web application so that it can be supported for a specific language or locale.
21) What is the difference between a page theme and a global theme?
Page Theme: The page theme is applied to particular web pages of the project. It is stored inside a subfolder of the App_Themes folder.
Global Theme: The Global theme is applied to all the web applications on the web server. It is stored inside the Themes folder on a Web server.
22) What is the difference between early binding and late binding?
Early Binding: In early binding, a non-virtual method is called which is decided at a compile time.
Late Binding: In late binding, a virtual method is called which is decided at runtime.
23) What is the difference between server-side scripting and client-side scripting?
Server-side scripting: In server-side scripting, all the script are executed by the server and interpreted as needed.
Client-side scripting: In client-side scripting, the script will be executed immediately in the browser such as form field validation, email validation, etc.The client-side scripting is usually carried out in VBScript or JavaScript.
Server-side scripting: In server-side scripting, all the script are executed by the server and interpreted as needed.
Client-side scripting: In client-side scripting, the script will be executed immediately in the browser such as form field validation, email validation, etc.The client-side scripting is usually carried out in VBScript or JavaScript.
24) How to sign out from forms authentication?
FormsAuthentication.Signout() method is used to sign out from forms authentication.
25) How to display validation messages in one control?
By the help of ValidationSummary control, we can display all validation messages in one control.
26) What is the difference between authentication and authorization?
Authentication is a process of identifying user whereas authorization is used to check the access rights of an identified user.
27) Which object encapsulates state or data of a user?
Session object.
28) What is ViewState?
ViewState is a feature of ASP.NET to store the values of a page before it is submitted to the server. After posting the page, data from is ViewState is restored.
Features of View State. These are the main features of view state:
1. Retains the value of the Control after post-back without using a session.
2. Stores the value of Pages and Control Properties defined in the page.
3. Creates a custom View State Provider that lets you store View State Information in a SQL Server Database or in another data store.
Advantages of View State
1. Easy to Implement.
2. No server resources are required: The View State is contained in a structure within the page load.
Enhanced security features: It can be encoded and compressed or Unicode implementation.
ViewState is a feature of ASP.NET to store the values of a page before it is submitted to the server. After posting the page, data from is ViewState is restored.
Features of View State. These are the main features of view state:
1. Retains the value of the Control after post-back without using a session.
2. Stores the value of Pages and Control Properties defined in the page.
3. Creates a custom View State Provider that lets you store View State Information in a SQL Server Database or in another data store.
Advantages of View State
1. Easy to Implement.
2. No server resources are required: The View State is contained in a structure within the page load.
Enhanced security features: It can be encoded and compressed or Unicode implementation.
29) What is ViewState information stored?
It is stored in HTML hidden field.
30) What are the differences between the Response.Write() and Response.Output.Write()?
Response.Write() is used for normal output whereas Response.Output.Write() is used for formatted output.
31) Define the types of configuration files.
There are two types of configuration files:
Application Level config = Web.config.
Machine Level config = Machine.config.
32) What is the difference between Web config and Machine config files?
Web config file is specific to web application whereas Machine config file is specific to machine or server.
There can be multiple web config files in an application but only one machine config file.
33) What is MVC?
MVC stands for Model View Controller. It is a design pattern that is used to separate business logic and presentation logic. It is used to develop the highly customized application.
The Model represents data, View represents presentation and controller acts as an interface between Model and View.
34) What are the built-in objects in ASP.NET?
The major built-in objects are given below:
Application
Session
Context
Request
Response
Server
Trace
35) What do you mean by Role-based security?
Role-based security is used in almost all organization, and the Role-based security assigns certain privileges to each role.
Each user is assigned a particular role from the list.
Privileges as per role restrict the user's actions on the system and ensure that a user can do only what he is permitted to do on the system.
Privileges as per role restrict the user's actions on the system and ensure that a user can do only what he is permitted to do on the system.
36) What is a cookie?
A Cookie is a small piece of information which is stored at the client side. There are two types of cookie:
Session/Temporary Cookie: valid for a single session
Persistent Cookie: valid for multiple session
Cookies are a State Management Technique that can store the values of control after a post-back. Cookies can store user-specific Information on the client's machine like when the user last visited your site. Cookies are also known by many names, such as HTTP Cookies, Browser Cookies, Web Cookies, Session Cookies and so on. Basically cookies are a small text file sent by the web server and saved by the Web Browser on the client's machine.
List of properties containing the HttpCookies Class:
Domain: Using these properties we can set the domain of the cookie.
Expires: This property sets the Expiration time of the cookies.
HasKeys: If the cookies have a subkey then it returns True.
Name: Contains the name of the Key.
Path: Contains the Virtual Path to be submitted with the Cookies.
Secured: If the cookies are to be passed in a secure connection then it only returns True.
Value: Contains the value of the cookies.
Limitation of the Cookies
The size of cookies is limited to 4096 bytes.
A total of 20 cookies can be used in a single website.
37) What is the default timeout for a cookie?
30 minutes.
38) How would you turn off cookies on a page of a website?
You have to follow the procedures given below:
Use the "Cookie.Discard" property.
It gets or sets the discard flag set by the server.
When set to true, this property instructs the client application not to save the Cookie on the hard disk of the user at the end of the session.
39) Which protocol is used to call web service?
HTTP protocol.
40) What is the file extension of web service?
The File extension of web service is .asmx.
41) What are the HTML server controls in ASP.NET?
HTML server controls are just like HTML elements that we use on the HTML pages.
HTML server controls are just like HTML elements that we use on the HTML pages.
HTML server controls are used to expose properties and events for use.
To make these controls programmatically accessible, we specify that the HTML controls act as a server control by adding the runat="server" attribute.
To make these controls programmatically accessible, we specify that the HTML controls act as a server control by adding the runat="server" attribute.
42) What is the use of Global.asax file?
The Global.asax file is used to execute the application-level events and sets application-level variables.
43) What is event bubbling?
When child control sends events to parent, it is termed as event bubbling. Server controls like Data Grid, Data List, and Repeater can have other child controls inside them.
44) What are the different validators in ASP.NET?
ASP.NET validation controls define an important role in validating the user input data. Whenever the user gives input, it must always be validated before sending it across the various layers of an application. There are two types of validation in ASP.NET:
Client-Side Validation
Server-Side Validation
Client-Side Validation: When validation is done on the client browser, it is known as Client-Side Validation. You can use JavaScript to do the Client-Side Validation.
Server-Side Validation: When validation occurs on the server, then it is known as Server-Side Validation. Server-Side Validation is a secure form of validation. The main advantage of Server-Side Validation is if the user bypasses the Client-Side Validation, the problem can be caught on the server-side.
The following are the Validation Controls in ASP.NET:
RequiredFieldValidator Control
CompareValidator Control
RangeValidator Control
RegularExpressionValidator Control
CustomFieldValidator Control
ValidationSummary
45) What is the difference between Server.Transfer and Response.Redirect?
In Server.Transfer page processing transfers from one page to the other page without making a round-trip back to the client's browser. This provides a faster response with a little less overhead on the server. The clients url history list or current url Server does not update in case of Server.Transfer.
Response.Redirect is used to redirect the user's browser to another page or site. It performs trip back to the client where the client's browser is redirected to the new page. The user's browser history list is updated to reflect the new address.
46) In which event of page cycle is the ViewState available?
After the Init() and before the Page_Load().
47) What are the different Session state management options available in ASP.NET?
In-Process
Out-of-Process.
In-Process stores the session in memory on the web server.
Out-of-Process Session state management stores data in an external server. The external server may be either a SQL Server or a State Server. All objects stored in session are required to be serializable for Out-of-Process state management.
48) How you can add an event handler?
Using the Attributes property of server side control.
btnSubmit.Attributes.Add("onMouseOver","JavascriptCode();")
49) What are the different types of caching?ASP.NET has 3 kinds of caching :
Output Caching,
Fragment Caching,
Data Caching.
50) List the events in page life cycle.
1) Page_PreInit
2) Page_Init
3) Page_InitComplete
4) Page_PreLoad
5) Page_Load
6) Page_LoadComplete
7) Page_PreRender
8) Render
ASP.NET goes through a series of stages in the life cycle of each page.
Page request. The user requests a page. ASP.NET decides whether to compile it or serve it from a cache.
Page Start. The Request and Response objects are created.
Page Initialization. All page controls are initialized, and any themes are applied.
Page Load. ASP.NET uses the view state and control state properties to set the control properties. Default values are set in the controls.
Postback event handling. This event is triggered if the same page is loaded again.
Rendering. ASP.NET saves the view state for the page and writes the output of rendering to the output stream. It happens just before the complete web page is sent to the user.
Unload. The rendered page gets sent to the client. ASP.NET unloads page properties and performs cleanup. All unwanted objects are removed from memory.
ASP.NET Page Life Cycle Events
Following are the page life cycle events:
PreInit
PreInit is the first event in page life cycle. It checks the IsPostBack property and determines whether the page is a postback. It sets the themes and master pages, creates dynamic controls and gets and sets profile property values. This event can be handled by overloading the OnPreInit method or creating a Page_PreInit handler.
Init
Init event initializes the control property and the control tree is built. This event can be handled by overloading the OnInit method or creating a Page_Init handler.
InitComplete
InitComplete event allows tracking of view state. All the controls turn on view-state tracking.
LoadViewState
LoadViewState event allows loading view state information into the controls.
LoadPostData
During this phase, the contents of all the input fields defined with the
51) What is Cross Page Posting?
When we click submit button on a web page, the page post the data to the same page. The technique in which we post the data to different pages is called Cross Page posting. This can be achieved by setting POSTBACKURL property of the button that causes the postback. Findcontrol method of PreviousPage can be used to get the posted values on the page to which the page has been posted.
ASP.NET 1.1 provides for web forms posting back only to themselves. In many situations, the solution requires posting to a different web page. The traditional workaround alternatives were to use Response.Redirect and/or Server.Transfer to move to a different page and simulate cross page post-back behavior.
ASP.NET 2.0 provides a feature known as Cross Page PostBack for a web form to post-back to a different web form (other than itself)
How to post to a different page
To set a web form to post back to a different web form, in the source web form, set the PostBackURL property of a control that implements IButtonControl (eg. Button, ImageButton, LinkButton) to the target web form. When the user clicks on this button control, the web form is cross-posted to the target web form. No other settings or code is required in the source web form.
Access source page info within the posted page: FindControl Method
The target web form resulting from the cross-page postback provides a non-null PreviousPage property. This property represents the source page and provides reference to the source web form and its controls.
The controls on the source page can be accessed via the FindControl method on the object returned by the PreviousPage property of the target page.
protected void Page_Load(object sender, EventArgs e)
{
...
TextBox txtStartDate = (TextBox) PreviousPage.FindControl("txtStartDate ");
...
}
At this point the target page does not have any knowledge of the source page. The PreviousPage property is of the type Page. For accessing controls using FindControl, the developer has to presume a certain structure in the source web form. This approach using FindControl has a few limitations. FindControl is dependent on the developer to provide the ids of the controls to access. The code will stop working if the control id is changed in the source web form. The FindControl method can retrieve controls only within the current container. If you need to access a control within another control, you need to first get a reference to the parent control.
Access source page info within the posted page: @PreviousPageType Directive
There is another more direct option to get access to the source page controls if the source page is pre-determined. The @PreviousPageType directive can be used in the target page to strongly type the source page. The directive specifies the source page using either the VirtualPath attribute or the TypeName attribute. The PreviousPage property then returns a strongly typed reference to the source page. It allows access to the public properties of the source page.
SourcePage.aspx:
TargetPage.aspx
<%@ PreviousPageType VirtualPath="sourcepage.aspx" %>
string strFirstName;
strFirstName = PreviousPage.FirstName //Strongly Typed PreviousPage allows direct access to the public properties of the source page.
52) How can we apply Themes to an asp.net application?
We can specify the theme in web.config file. Below is the code example to apply theme:
We can specify the theme in web.config file. Below is the code example to apply theme:
53) What is RedirectPermanent in ASP.Net?
RedirectPermanent Performs a permanent redirection from the requested URL to the specified URL. Once the redirection is done, it also returns 301 Moved Permanently responses.
RedirectPermanent Performs a permanent redirection from the requested URL to the specified URL. Once the redirection is done, it also returns 301 Moved Permanently responses.
54) Explain the working of passport authentication.
First of all it checks passport authentication cookie. If the cookie is not available then the application redirects the user to Passport Sign on page. Passport service authenticates the user details on sign on page and if valid then stores the authenticated cookie on client machine and then redirect the user to requested page
55) What are the advantages of Passport authentication?
All the websites can be accessed using single login credentials. So no need to remember login credentials for each web site.
First of all it checks passport authentication cookie. If the cookie is not available then the application redirects the user to Passport Sign on page. Passport service authenticates the user details on sign on page and if valid then stores the authenticated cookie on client machine and then redirect the user to requested page
55) What are the advantages of Passport authentication?
All the websites can be accessed using single login credentials. So no need to remember login credentials for each web site.
Users can maintain his/ her information in a single location.
Boxing is assigning a value type to reference type variable.
Unboxing is reverse of boxing ie. Assigning reference type variable to value type variable.
57) What are the components of ADO.NET?
The components of ADO.Net are Dataset, Data Reader, Data Adaptor, Command, connection.
58) What is the difference between ExecuteScalar and ExecuteNonQuery?
ExecuteScalar returns output value where as ExecuteNonQuery does not return any value but the number of rows affected by the query. ExecuteScalar used for fetching a single value and ExecuteNonQuery used to execute Insert and Update statements.
59) Which data type does the RangeValidator control support?
The data types supported by the RangeValidator control are Integer, Double, String, Currency, and Date.
60) What is the difference between an HtmlInputCheckBox control and an HtmlInputRadioButton control?
In HtmlInputCheckBoxcontrol, multiple item selection is possible whereas in HtmlInputRadioButton controls, we can select only single item from the group of items.
61) Which namespaces are necessary to create a localized application?
System.Globalization
System.Resources
62) What is the appSettings Section in the web.config file?
The appSettings block in web config file sets the user-defined values for the whole application.
For example, in the following code snippet, the specified ConnectionString section is used throughout the project for database connection:
The appSettings block in web config file sets the user-defined values for the whole application.
For example, in the following code snippet, the specified ConnectionString section is used throughout the project for database connection:
63) List all templates of the Repeater control.
ItemTemplate
AlternatingltemTemplate
SeparatorTemplate
HeaderTemplate
FooterTemplate
Page load event.
65) What is boxing and unboxing?
Boxing is assigning a value type to reference type variable.
Unboxing is reverse of boxing ie. Assigning reference type variable to value type variable.
66) Differentiate strong typing and weak typing
In strong typing, the data types of variable are checked at compile time. On the other hand, in case of weak typing the variable data types are checked at runtime. In case of strong typing, there is no chance of compilation error. Scripts use weak typing and hence issues arises at runtime.
67) How we can force all the validation controls to run?
The Page.Validate() method is used to force all the validation controls to run and to perform validation.
68) What is the difference between custom controls and user controls?
Custom controls are basically compiled code, i.e., DLLs. These can be easily added to the toolbox, so it can be easily used across multiple projects using a drag-and-drop approach. These controls are comparatively hard to create. But User Controls (.ascx) are just like pages (.aspx). These are comparatively easy to create but tightly coupled with respect to User Interface and code.
69) What are the types of Authentication in ASP.NET?
There are three types of authentication available in ASP.NET:
Windows Authentication: This authentication method uses built-in Windows security features to authenticate a user.
Forms Authentication: Authenticates against a customized list of users or users in a database.
Passport Authentication: Validates against Microsoft Passport service which is basically a centralized authentication service.
Custom controls are basically compiled code, i.e., DLLs. These can be easily added to the toolbox, so it can be easily used across multiple projects using a drag-and-drop approach. These controls are comparatively hard to create. But User Controls (.ascx) are just like pages (.aspx). These are comparatively easy to create but tightly coupled with respect to User Interface and code.
69) What are the types of Authentication in ASP.NET?
There are three types of authentication available in ASP.NET:
Windows Authentication: This authentication method uses built-in Windows security features to authenticate a user.
Forms Authentication: Authenticates against a customized list of users or users in a database.
Passport Authentication: Validates against Microsoft Passport service which is basically a centralized authentication service.
70) What is ASP.NET AJAX?
Ajax stands for Asynchronous JavaScript and XML; in other words Ajax is the combination of various technologies such as a JavaScript, CSS, XHTML, DOM, etc.
AJAX allows web pages to be updated asynchronously by exchanging small amounts of data with the server behind the scenes. This means that it is possible to update parts of a web page, without reloading the entire page.
We can also define Ajax is a combination of client side technologies that provides asynchronous communication between the user interface and the web server so that partial page rendering occurs instead of complete page post back.
Ajax is platform-independent; in other words AJAX is a cross-platform technology that can be used on any Operating System since it is based on XML & JavaScript. It also supports open source implementation of other technology. It partially renders the page to the server instead of complete page post back. We use AJAX for developing faster, better and more interactive web applications.
AJAX uses a HTTP request between web server & browser.
With AJAX, when a user clicks a button, you can use JavaScript and DHTML to immediately update the UI, and spawn an asynchronous request to the server to fetch results.
When the response is generated, you can then use JavaScript and CSS to update your UI accordingly without refreshing the entire page. While this is happening, the form on the users screen doesn't flash, blink, disappear, or stall.
The power of AJAX lies in its ability to communicate with the server asynchronously, using a XMLHttpRequest object without requiring a browser refresh.
Ajax essentially puts JavaScript technology and the XMLHttpRequest object between your Web form and the server.
71) What is the REST architecture?
REST (Representational State Transfer) is an architectural style for designing applications and it dictates to use HTTP for making calls for communications instead of complex mechanism like CORBA, RPC or SOAP.
REST (Representational State Transfer) is an architectural style for designing applications and it dictates to use HTTP for making calls for communications instead of complex mechanism like CORBA, RPC or SOAP.
There are few principles associated with REST architectural style:
Everything is a resource i.e. File, Images, Video, WebPage etc.
Every Resource is identified by a Unique Identifier.
Use simple and Uniform Interfaces.
Everything is done via representation (sending requests from a client to server and receiving responses from server to client).
Be Stateless- Every request should be an independent request.
72) Which compiler is used in ASP.NET?
Answer: Roslyn is the name of the compiler used by .NET Framework.
73) What are the different Session state management options available in ASP.NET?
State Management in ASP.NET?
A new instance of the Web page class is created each time the page is posted to the server.
In traditional Web programming, all information that is associated with the page, along with the controls on the page, would be lost with each roundtrip.
The Microsoft ASP.NET framework includes several options to help you preserve data on both a per-page basis and an application-wide basis.
These options can be broadly divided into the following two categories:
Client-Side State Management Options
Server-Side State Management Options
Client-Side State Management
Client-based options involve storing information either in the page or on the client computer.
Some client-based state management options are:
Hidden fields
View state
Cookies
Query strings
Server-Side State Management
There are situations where you need to store the state information on the server side.
Server-side state management enables you to manage application-related and session-related information on the server.
ASP.NET provides the following options to manage state at the server side:
Application state
Session state
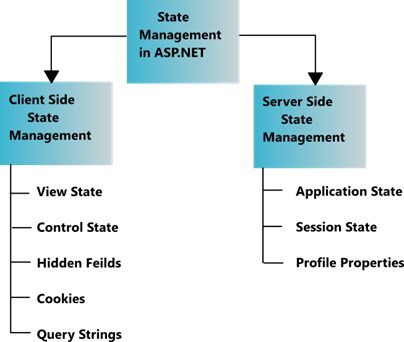
74) What are Web Services in ASP.NET?
A Web Service is a software program that uses XML to exchange information with other software via common internet protocols. In a simple sense, Web Services are a way for interacting with objects over the Internet.
A web service is:
Language Independent.
Protocol Independent.
Platform Independent.
It assumes a stateless service architecture.
Scalable (e.g. multiplying two numbers together to an entire customer-relationship management system).
Programmable (encapsulates a task).
Based on XML (open, text-based standard).
Self-describing (metadata for access and use).
Discoverable (search and locate in registries)- ability of applications and developers to search for and locate desired Web services through registries. This is based on UDDI.
Key Web Service Technologies:
XML- Describes only data. So, any application that understands XML-regardless of the application's programming language or platform-has the ability to format XML in a variety of ways (well-formed or valid).
SOAP- Provides a communication mechanism between services and applications.
WSDL- Offers a uniform method of describing web services to other programs.
UDDI- Enables the creation of searchable Web services registries.
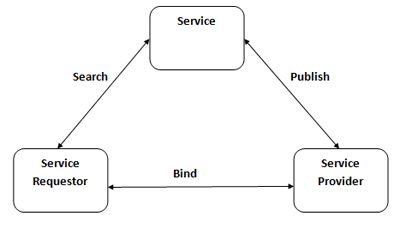
Every Resource is identified by a Unique Identifier.
Use simple and Uniform Interfaces.
Everything is done via representation (sending requests from a client to server and receiving responses from server to client).
Be Stateless- Every request should be an independent request.
72) Which compiler is used in ASP.NET?
Answer: Roslyn is the name of the compiler used by .NET Framework.
73) What are the different Session state management options available in ASP.NET?
State Management in ASP.NET?
A new instance of the Web page class is created each time the page is posted to the server.
In traditional Web programming, all information that is associated with the page, along with the controls on the page, would be lost with each roundtrip.
The Microsoft ASP.NET framework includes several options to help you preserve data on both a per-page basis and an application-wide basis.
These options can be broadly divided into the following two categories:
Client-Side State Management Options
Server-Side State Management Options
Client-Side State Management
Client-based options involve storing information either in the page or on the client computer.
Some client-based state management options are:
Hidden fields
View state
Cookies
Query strings
Server-Side State Management
There are situations where you need to store the state information on the server side.
Server-side state management enables you to manage application-related and session-related information on the server.
ASP.NET provides the following options to manage state at the server side:
Application state
Session state
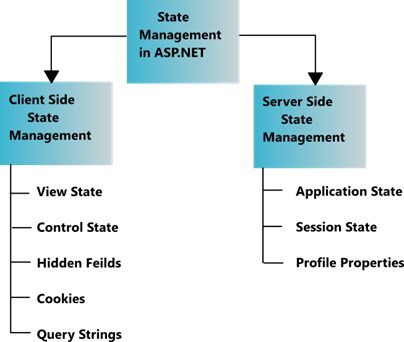
74) What are Web Services in ASP.NET?
A Web Service is a software program that uses XML to exchange information with other software via common internet protocols. In a simple sense, Web Services are a way for interacting with objects over the Internet.
A web service is:
Language Independent.
Protocol Independent.
Platform Independent.
It assumes a stateless service architecture.
Scalable (e.g. multiplying two numbers together to an entire customer-relationship management system).
Programmable (encapsulates a task).
Based on XML (open, text-based standard).
Self-describing (metadata for access and use).
Discoverable (search and locate in registries)- ability of applications and developers to search for and locate desired Web services through registries. This is based on UDDI.
Key Web Service Technologies:
XML- Describes only data. So, any application that understands XML-regardless of the application's programming language or platform-has the ability to format XML in a variety of ways (well-formed or valid).
SOAP- Provides a communication mechanism between services and applications.
WSDL- Offers a uniform method of describing web services to other programs.
UDDI- Enables the creation of searchable Web services registries.
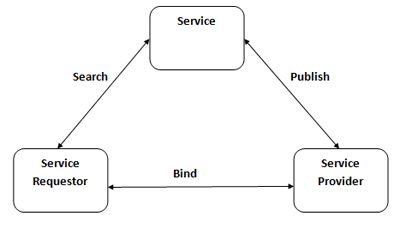
75) What is the App Domain Concept in ASP.NET?
ASP.NET introduces the concept of an Application Domain which is shortly known as AppDomain. It can be considered as a Lightweight process which is both a container and boundary. The .NET runtime uses an AppDomain as a container for code and data, just like the operating system uses a process as a container for code and data. As the operating system uses a process to isolate misbehaving code, the .NET runtime uses an AppDomain to isolate code inside a secure boundary.
The CLR can allow the multiple .NET applications to run in a single AppDomain. Mulitple Appdomains can exist in Win32 process.
How to create AppDomain: AppDomains are created using the CreateDomain method. AppDomain instances are used to load and execute assemblies (Assembly). When an AppDomain is no longer in use, it can be unloaded.
76) What is master page in ASP.NET?
The extension of MasterPage is '.master'. MasterPage cannot be directly accessed from the client because it just acts as a template for the other Content Pages. In a MasterPage we can have content either inside ContentPlaceHolder or outside it. Only content inside the ContentPlaceHolder can be customized in the Content Page. We can have multiple masters in one web application.A MasterPage can have another MasterPage as Master to it. The MasterPageFile property of a webform can be set dynamically and it should be done either in or before the Page_PreInit event of the WebForm. Page.MasterPageFile = "MasterPage.master". The dynamically set Master Page must have the ContentPlaceHolder whose content has been customized in the WebForm.
Some points about Master Pages:
1. The extension of MasterPage is '.master'.
2. MasterPage cannot be directly accessed from the client because it just acts as a template for the other Content Pages.
3. In a MasterPage we can have content either inside ContentPlaceHolder or outside it. Only content inside the ContentPlaceHolder can be customized in the Content Page.
4. We can have multiple masters in one web application.
ASP.NET introduces the concept of an Application Domain which is shortly known as AppDomain. It can be considered as a Lightweight process which is both a container and boundary. The .NET runtime uses an AppDomain as a container for code and data, just like the operating system uses a process as a container for code and data. As the operating system uses a process to isolate misbehaving code, the .NET runtime uses an AppDomain to isolate code inside a secure boundary.
The CLR can allow the multiple .NET applications to run in a single AppDomain. Mulitple Appdomains can exist in Win32 process.
How to create AppDomain: AppDomains are created using the CreateDomain method. AppDomain instances are used to load and execute assemblies (Assembly). When an AppDomain is no longer in use, it can be unloaded.
- public class MyAppDomain: MarshalByRefObject
- {
- public string GetInfo()
- {
- return AppDomain.CurrentDomain.FriendlyName;
- }
- }
- public class MyApp
- {
- public static void Main()
- {
- AppDomain apd = AppDomain.CreateDomain("Rajendrs Domain");
- MyAppDomain apdinfo = (MyAppDomain) apd.CreateInstanceAndUnwrap(Assembly.GetCallingAssembly()
- .GetName()
- .Name, "MyAppDomain");
- Console.WriteLine("Application Name = " + apdinfo.GetInfo());
- }
- }
76) What is master page in ASP.NET?
The extension of MasterPage is '.master'. MasterPage cannot be directly accessed from the client because it just acts as a template for the other Content Pages. In a MasterPage we can have content either inside ContentPlaceHolder or outside it. Only content inside the ContentPlaceHolder can be customized in the Content Page. We can have multiple masters in one web application.A MasterPage can have another MasterPage as Master to it. The MasterPageFile property of a webform can be set dynamically and it should be done either in or before the Page_PreInit event of the WebForm. Page.MasterPageFile = "MasterPage.master". The dynamically set Master Page must have the ContentPlaceHolder whose content has been customized in the WebForm.
Some points about Master Pages:
1. The extension of MasterPage is '.master'.
2. MasterPage cannot be directly accessed from the client because it just acts as a template for the other Content Pages.
3. In a MasterPage we can have content either inside ContentPlaceHolder or outside it. Only content inside the ContentPlaceHolder can be customized in the Content Page.
4. We can have multiple masters in one web application.
5. A MasterPage can have another MasterPage as Master to it.
6. The content page content can be placed only inside the content tag.
7. Controls of MasterPage can be programmed in the MasterPage and content page but a content page control will never be programmed in MasterPage.
8. A master page of one web application cannot be used in another web application.
9. The MasterPageFile property of a webform can be set dynamically and it should be done either in or before the Page_PreInit event of the WebForm. Page.MasterPageFile = "MasterPage.master". The dynamically set Master Page must have the ContentPlaceHolder whose content has been customized in the WebForm.
10. The order in which events are raised: Load (Page) a Load (Master) a LoadComplete (Page) i.e. if we want to overwrite something already done in Load event handler of Master then it should be coded in the LoadComplete event of the page.
11. Page_Load is the name of method for event handler for Load event of Master. (it's not Master_Load)
A master page is defined using the following code:
<%@ master language="C#" %>
Adding a MasterPage to the Project
1 Add a new MasterPage file (MainMaster.master) to the Web Application.
2 Change the Id of ContentPlaceHolder in to "cphHead" and the Id "ContentPlaceHolder1" to "cphFirst".
3 Add one more ContentPlaceHolder (cphSecond) to Master page.
4 To the master page add some header, footer and some default content for both the content place holders.
This is First Content Place Holder (Default)
6. The content page content can be placed only inside the content tag.
7. Controls of MasterPage can be programmed in the MasterPage and content page but a content page control will never be programmed in MasterPage.
8. A master page of one web application cannot be used in another web application.
9. The MasterPageFile property of a webform can be set dynamically and it should be done either in or before the Page_PreInit event of the WebForm. Page.MasterPageFile = "MasterPage.master". The dynamically set Master Page must have the ContentPlaceHolder whose content has been customized in the WebForm.
10. The order in which events are raised: Load (Page) a Load (Master) a LoadComplete (Page) i.e. if we want to overwrite something already done in Load event handler of Master then it should be coded in the LoadComplete event of the page.
11. Page_Load is the name of method for event handler for Load event of Master. (it's not Master_Load)
A master page is defined using the following code:
<%@ master language="C#" %>
Adding a MasterPage to the Project
1 Add a new MasterPage file (MainMaster.master) to the Web Application.
2 Change the Id of ContentPlaceHolder in to "cphHead" and the Id "ContentPlaceHolder1" to "cphFirst".
3 Add one more ContentPlaceHolder (cphSecond) to Master page.
4 To the master page add some header, footer and some default content for both the content place holders.
This is Second Content Place Holder (Default).
Footer...
77) What are the data controls available in ASP.NET?
The Controls having DataSource Property are called Data Controls in ASP.NET. ASP.NET allows powerful feature of data binding, you can bind any server control to simple properties, collections, expressions and/or methods. When you use data binding, you have more flexibility when you use data from a database or other means.
Data Bind controls are container controls.
Controls -> Child Control
Data Binding is binding controls to data from databases. With data binding we can bind a control to a particular column in a table from the database or we can bind the whole table to the data grid.
Data binding provides simple, convenient, and powerful way to create a read/write link between the controls on a form and the data in their application.
Data binding allows you to take the results of properties, collection, method calls, and database queries and integrate them with your ASP.NET code. You can combine data binding with Web control rendering to relieve much of the programming burden surrounding Web control creation. You can also use data binding with ADO.NET and Web controls to populate control contents from SQL select statements or stored procedures.
Data binding uses a special syntax:
<%# %>
The <%#, which instructs ASP.NET to evaluate the expression. The difference between a data binding tags and a regular code insertion tags <% and %> becomes apparent when the expression is evaluated. Expressions within the data binding tags are evaluated only when the DataBind method in the Page objects or Web control is called.
Data Bind Control can display data in connected and disconnected model.
Following are data bind controls in ASP.NET:
Repeater Control
DataGrid Control
DataList Control
GridView Control
DetailsView
FormView
DropDownList
ListBox
RadioButtonList
CheckBoxList
BulletList etc.
The Controls having DataSource Property are called Data Controls in ASP.NET. ASP.NET allows powerful feature of data binding, you can bind any server control to simple properties, collections, expressions and/or methods. When you use data binding, you have more flexibility when you use data from a database or other means.
Data Bind controls are container controls.
Controls -> Child Control
Data Binding is binding controls to data from databases. With data binding we can bind a control to a particular column in a table from the database or we can bind the whole table to the data grid.
Data binding provides simple, convenient, and powerful way to create a read/write link between the controls on a form and the data in their application.
Data binding allows you to take the results of properties, collection, method calls, and database queries and integrate them with your ASP.NET code. You can combine data binding with Web control rendering to relieve much of the programming burden surrounding Web control creation. You can also use data binding with ADO.NET and Web controls to populate control contents from SQL select statements or stored procedures.
Data binding uses a special syntax:
<%# %>
The <%#, which instructs ASP.NET to evaluate the expression. The difference between a data binding tags and a regular code insertion tags <% and %> becomes apparent when the expression is evaluated. Expressions within the data binding tags are evaluated only when the DataBind method in the Page objects or Web control is called.
Data Bind Control can display data in connected and disconnected model.
Following are data bind controls in ASP.NET:
Repeater Control
DataGrid Control
DataList Control
GridView Control
DetailsView
FormView
DropDownList
ListBox
RadioButtonList
CheckBoxList
BulletList etc.
78) What is page directives in ASP.NET?
Basically Page Directives are commands. These commands are used by the compiler when the page is compiled.
How to use the directives in an ASP.NET page
It is not difficult to add a directive to an ASP.NET page. It is simple to add directives to an ASP.NET page. You can write directives in the following format:
<%@[Directive][Attributes]%>
See the directive format, it starts with "<%@" and ends with "%>". The best way is to put the directive at the top of your page. But you can put a directive anywhere in a page. One more thing, you can put more than one attribute in a single directive.
Here is the full list of directives:
@Page
@Master
@Control
@Import
@Implements
@Register
@Assembly
@MasterType
@Output Cache
@PreviousPageType
@Reference
79) What is HTTP Handler?
Every request into an ASP.NET application is handled by a specialized component known as an HTTP handler. The HTTP handler is the most important ingredient while handling ASP.NET requests.
Examples: ASP.NET uses different HTTP handlers to serve different file types. For example, the handler for web Page creates the page and control objects, runs your code, and renders the final HTML.
ASP.NET default handlers:
1. Page Handler (.aspx) - Handles Web pages.
2. User Control Handler (.ascx) - Handles Web user control pages.
3. Web Service Handler (.asmx) - Handles Web service pages.
4. Trace Handler (trace.axd) - Handles trace functionality.
Why we need to create our own HTTP Handler: Sometime we need to avoid ASP.NET full page processing model, which saves lot of overheads, as ASP.NET web form model has to go through many steps such as creating web page objects, persisting view state etc. What we are interested into is to develop some low level interface that provides access to objects like Request and Response but doesn't use the full control based web form model discussed above.
Examples:
1. Dynamic image creator - Use the System.Drawing classes to draw and size your own images.
2. RSS - Create a handler that responds with RSS-formatted XML. This would allow you to add RSS feed capabilities to your sites.
3. Render a custom image,
4. Perform an ad hoc database query,
4. Return some binary data.
All HTTP handlers are defined in the section of a configuration file which is nested in the element.
Basically Page Directives are commands. These commands are used by the compiler when the page is compiled.
How to use the directives in an ASP.NET page
It is not difficult to add a directive to an ASP.NET page. It is simple to add directives to an ASP.NET page. You can write directives in the following format:
<%@[Directive][Attributes]%>
See the directive format, it starts with "<%@" and ends with "%>". The best way is to put the directive at the top of your page. But you can put a directive anywhere in a page. One more thing, you can put more than one attribute in a single directive.
Here is the full list of directives:
@Page
@Master
@Control
@Import
@Implements
@Register
@Assembly
@MasterType
@Output Cache
@PreviousPageType
@Reference
79) What is HTTP Handler?
Every request into an ASP.NET application is handled by a specialized component known as an HTTP handler. The HTTP handler is the most important ingredient while handling ASP.NET requests.
Examples: ASP.NET uses different HTTP handlers to serve different file types. For example, the handler for web Page creates the page and control objects, runs your code, and renders the final HTML.
ASP.NET default handlers:
1. Page Handler (.aspx) - Handles Web pages.
2. User Control Handler (.ascx) - Handles Web user control pages.
3. Web Service Handler (.asmx) - Handles Web service pages.
4. Trace Handler (trace.axd) - Handles trace functionality.
Why we need to create our own HTTP Handler: Sometime we need to avoid ASP.NET full page processing model, which saves lot of overheads, as ASP.NET web form model has to go through many steps such as creating web page objects, persisting view state etc. What we are interested into is to develop some low level interface that provides access to objects like Request and Response but doesn't use the full control based web form model discussed above.
Examples:
1. Dynamic image creator - Use the System.Drawing classes to draw and size your own images.
2. RSS - Create a handler that responds with RSS-formatted XML. This would allow you to add RSS feed capabilities to your sites.
3. Render a custom image,
4. Perform an ad hoc database query,
4. Return some binary data.
All HTTP handlers are defined in the
80) What are Differences between ASP.NET HttpHandler and HttpModule?
The user requests for a resource on web server. The web server examines the file name extension of the requested file, and determines which ISAPI extension should handle the request. Then the request is passed to the appropriate ISAPI extension. For example when an .aspx page is requested it is passed to ASP.NET page handler. Then Application domain is created and after that different ASP.NET objects like Httpcontext, HttpRequest, HttpResponse are created. Then instance of HttpApplication is created and also instance of any configured modules. One can register different events of HttpApplication class like BeginRequest, AuthenticateRequest, AuthorizeRequest, ProcessRequest etc.
HTTP Handler
HTTP Handler is the process which runs in response to a HTTP request. So whenever user requests a file it is processed by the handler based on the extension. So, custom http handlers are created when you need to special handling based on the file name extension. Let's consider an example to create RSS for a site. So, create a handler that generates RSS-formatted XML. Now bind the .rss extension to the custom handler.
HTTP Modules
HTTP Modules are plugged into the life cycle of a request. So when a request is processed it is passed through all the modules in the pipeline of the request. So generally http modules are used for:
Security: For authenticating a request before the request is handled.
Statistics and Logging: Since modules are called for every request they can be used for gathering statistics and for logging information.
Custom header: Since response can be modified, one can add custom header information to the response.
81) Explain the AdRotator Control?
AdRotator control are used to create a dynamic ads. The AdRotator Control presents ad images each time a user enters or refreshes a webpage. When the ads are clicked, it will navigate to a new Web location. The AdRotator control is used to display a sequence of ad images.The AdRotator control to work we need an Advertisement file (XML file) and some sample images.
Adding the AdRotator web server control to your web application. first, select the AdRotator and drag and drop the control to your web form. Map the XML file which contains the details about each and every ad.
The advertisement file is an XML file. The following are some of the elements of this XML file.
1.
2.
3.
4.
XML code that has the details about the ads. The file Ads.xml looks like the code below:
The GridView control displays the values of a data source in a table. Each column represents a field, while each row represents a record. The GridView control supports the following features:
Binding to data source controls, such as SqlDataSource.
Built-in sort capabilities.
Built-in update and delete capabilities.
Built-in paging capabilities.
Built-in row selection capabilities.
Programmatic access to the GridView object model to dynamically set properties, handle events, and so on.
Multiple key fields.
Multiple data fields for the hyperlink columns.
Customizable appearance through themes and styles.
Creating a GridView
Built-in sort capabilities.
Built-in update and delete capabilities.
Built-in paging capabilities.
Built-in row selection capabilities.
Programmatic access to the GridView object model to dynamically set properties, handle events, and so on.
Multiple key fields.
Multiple data fields for the hyperlink columns.
Customizable appearance through themes and styles.
Creating a GridView
83) Explain Cookie-less Session in ASP.NET?
By default a session uses a cookie in the background. To enable a cookie-less session, we need to change some configuration in the Web.Config file. Follow these steps:
1. Open Web.Config file.
2. Add a tag under tag.
3. Add an attribute "cookieless" in the tag and set its value to "AutoDetect" like below:
The possible values for "cookieless" attribute are:
AutoDetect: Session uses background cookie if cookies are enabled. If cookies are disabled, then the URL is used to store session information.
UseCookie: Session always use background cookie. This is default.
UseDeviceProfile: Session uses background cookie if browser supports cookies else URL is used.
UseUri: Session always use URL.
"regenerateExpiredSessionId" is used to ensure that if a cookieless url is expired a new new url is created with a new session. And if the same cookieless url is being used by multiple users an the same time, they all get a new regenerated session url.
84) What is Themes in ASP.NET?
A theme decides the look and feel of the website. It is a collection of files that define the looks of a page. It can include skin files, CSS files & images.
We define themes in a special App_Themes folder. Inside this folder is one or more subfolders named Theme1, Theme2 etc. that define the actual themes. The theme property is applied late in the page's life cycle, effectively overriding any customization you may have for individual controls on your page.
How to apply themes
There are 3 different options to apply themes to our website:
1. Setting the theme at the page level: the Theme attribute is added to the page directive of the page.
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs"Inherits="Default" Theme="Theme1"%>
2. Setting the theme at the site level: to set the theme for the entire website you can set the theme in the web.config of the website. Open the web.config file and locate the element and add the theme attribute to it:
....
....
3. Setting the theme programmatically at runtime: here the theme is set at runtime through coding. It should be applied earlier in the page's life cycle ie. Page_PreInit event should be handled for setting the theme. The better option is to apply this to the Base page class of the site as every page in the site inherits from this class.
Page.Theme = Theme1;
Uses of Themes
1. Since themes can contain CSS files, images and skins, you can change colors, fonts, positioning and images simply by applying the desired themes.
2. You can have as many themes as you want and you can switch between them by setting a single attribute in the web.config file or an individual aspx page. Also you can switch between themes programmatically.
3. Setting the themes programmatically, you are offering your users a quick and easy way to change the page to their likings.
4. Themes allow you to improve the usability of your site by giving users with vision problems the option to select a high contrast theme with a large font size.
85) What is WebParts in ASP.NET?
ASP.NET 2.0 incorporates the concept of WEB PARTS in itself and we can code and explore that as easily as we have done with the other controls in the previous sessions.
We can compose web parts pages from "web parts", which can be web controls, user controls.
Component of Web Parts: The web parts consist of different components like:
Web Part Manager
Web Part Zone
CatLog Part
CatLog Zone
Connections Zone
Editor Part
Editor Zone
Web Part Zone
Web Part Zone can contain one or more Web Part controls.
This provides the layout for the Controls it contains. A single ASPX page can contain one or more Web Part Zones.
A Web Part Control can be any of the controls in the toolbox or even the customized user controls.
86) How can we improve the Performance of an ASP.NET Web Page?
This is the most common question from ASP.NET forum to any interview. In this post I’m going to point out some of the important points that may help to improve the performance.
Here I used the word “improve performance” in the sense to decrease the loading time of the page. There are various reasons behind. Some of them we look into from the “backend side” (Database side) and rest of them we need to take care in “front-end” ((UI) side.
For illustrative purpose, you have an ASP.NET Web site, one of the aspx page take much time to load. Throughout this article, we are going to see how to decrease the loading time.
Back End (DB)
1. Try to check the Query performance that is how much time the query will take to execute and pull the records from DB. Then use SQL Server Profiler and Execution plan for that query so that you can come to a conclusion in which part it took much time.
2, Check in every table (who are all part of the query) Index is created properly.
3. If your query involves a complex stored procedure, which in turn use lot of joins, then you should focus on every table. In some cases, sub-query perform better than the joins.
4. If your web page involves paging concepts, try to move the paging concepts to SQL Server. I meant that based on the page count the SP will return the records, instead of bringing the records as a whole.
87) What are the major events in global.aspx?
The Global.asax file, which is derived from the HttpApplication class, maintains a pool of HttpApplication objects, and assigns them to applications as needed. The Global.asax file contains the following events:
Application_Init
Application_Disposed
Application_Error
Application_Start
Application_End
Application_BeginReques
By default a session uses a cookie in the background. To enable a cookie-less session, we need to change some configuration in the Web.Config file. Follow these steps:
1. Open Web.Config file.
2. Add a
3. Add an attribute "cookieless" in the
AutoDetect: Session uses background cookie if cookies are enabled. If cookies are disabled, then the URL is used to store session information.
UseCookie: Session always use background cookie. This is default.
UseDeviceProfile: Session uses background cookie if browser supports cookies else URL is used.
UseUri: Session always use URL.
"regenerateExpiredSessionId" is used to ensure that if a cookieless url is expired a new new url is created with a new session. And if the same cookieless url is being used by multiple users an the same time, they all get a new regenerated session url.
84) What is Themes in ASP.NET?
A theme decides the look and feel of the website. It is a collection of files that define the looks of a page. It can include skin files, CSS files & images.
We define themes in a special App_Themes folder. Inside this folder is one or more subfolders named Theme1, Theme2 etc. that define the actual themes. The theme property is applied late in the page's life cycle, effectively overriding any customization you may have for individual controls on your page.
How to apply themes
There are 3 different options to apply themes to our website:
1. Setting the theme at the page level: the Theme attribute is added to the page directive of the page.
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs"Inherits="Default" Theme="Theme1"%>
2. Setting the theme at the site level: to set the theme for the entire website you can set the theme in the web.config of the website. Open the web.config file and locate the
....
....
3. Setting the theme programmatically at runtime: here the theme is set at runtime through coding. It should be applied earlier in the page's life cycle ie. Page_PreInit event should be handled for setting the theme. The better option is to apply this to the Base page class of the site as every page in the site inherits from this class.
Page.Theme = Theme1;
Uses of Themes
1. Since themes can contain CSS files, images and skins, you can change colors, fonts, positioning and images simply by applying the desired themes.
2. You can have as many themes as you want and you can switch between them by setting a single attribute in the web.config file or an individual aspx page. Also you can switch between themes programmatically.
3. Setting the themes programmatically, you are offering your users a quick and easy way to change the page to their likings.
4. Themes allow you to improve the usability of your site by giving users with vision problems the option to select a high contrast theme with a large font size.
85) What is WebParts in ASP.NET?
ASP.NET 2.0 incorporates the concept of WEB PARTS in itself and we can code and explore that as easily as we have done with the other controls in the previous sessions.
We can compose web parts pages from "web parts", which can be web controls, user controls.
Component of Web Parts: The web parts consist of different components like:
Web Part Manager
Web Part Zone
CatLog Part
CatLog Zone
Connections Zone
Editor Part
Editor Zone
Web Part Zone
Web Part Zone can contain one or more Web Part controls.
This provides the layout for the Controls it contains. A single ASPX page can contain one or more Web Part Zones.
A Web Part Control can be any of the controls in the toolbox or even the customized user controls.
86) How can we improve the Performance of an ASP.NET Web Page?
This is the most common question from ASP.NET forum to any interview. In this post I’m going to point out some of the important points that may help to improve the performance.
Here I used the word “improve performance” in the sense to decrease the loading time of the page. There are various reasons behind. Some of them we look into from the “backend side” (Database side) and rest of them we need to take care in “front-end” ((UI) side.
For illustrative purpose, you have an ASP.NET Web site, one of the aspx page take much time to load. Throughout this article, we are going to see how to decrease the loading time.
Back End (DB)
1. Try to check the Query performance that is how much time the query will take to execute and pull the records from DB. Then use SQL Server Profiler and Execution plan for that query so that you can come to a conclusion in which part it took much time.
2, Check in every table (who are all part of the query) Index is created properly.
3. If your query involves a complex stored procedure, which in turn use lot of joins, then you should focus on every table. In some cases, sub-query perform better than the joins.
4. If your web page involves paging concepts, try to move the paging concepts to SQL Server. I meant that based on the page count the SP will return the records, instead of bringing the records as a whole.
87) What are the major events in global.aspx?
The Global.asax file, which is derived from the HttpApplication class, maintains a pool of HttpApplication objects, and assigns them to applications as needed. The Global.asax file contains the following events:
Application_Init
Application_Disposed
Application_Error
Application_Start
Application_End
Application_BeginReques
88) What is the authentication and authorization in ASP.NET?
Authentication: Prove genuineness
Authorization: process of granting approval or permission on resources.
In ASP.NET Authentication means to identify the user or in other words its nothing but to validate that he exists in your database and he is the proper user.
Authorization means does he have access to a particular resource on the IIS website. A resource can be an ASP.NET web page, media files (MP4, GIF, JPEG etc), compressed file (ZIP, RAR) etc.
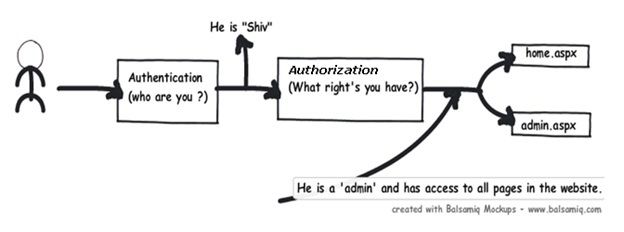
Types of authentication and authorization in ASP.NET
There are three ways of doing authentication and authorization in ASP.NET:
Windows authentication: In this methodology ASP.NET web pages will use local windows users and groups to authenticate and authorize resources.
Forms Authentication: This is a cookie based authentication where username and password are stored on client machines as cookie files or they are sent through URL for every request. Form-based authentication presents the user with an HTML-based Web page that prompts the user for credentials.
Passport authentication: Passport authentication is based on the passport website provided by the Microsoft .So when user logins with credentials it will be reached to the passport website ( i.e. hotmail,devhood,windows live etc) where authentication will happen. If Authentication is successful it will return a token to your website.
Anonymous access: If you do not want any kind of authentication then you will go for Anonymous access.
In 'web.config' file set the authentication mode to 'Windows' as shown in the below code snippets.
We also need to ensure that all users are denied except authorized users. The below code snippet inside the authorization tag that all users are denied. '?' indicates any unknown user.
89) Describe application state management in ASP.NET?
Application Level State Management is used to maintain the state of all the users accessing the web forms present within the website.
The value assigned for an application is considered as an object.
Application object will not have any default expiration period.
Whenever the webserver has been restarted or stopped then the information maintained by the application object will be lost.
If any data is stored on the application object then that information will be shared upon all the users accessing the webserver.
Since the information is shared among all the users, it is advisable to lock and unlock the application object as per requirement.
Global Application Class(Global.asax):
It is a Class which consists of event handlers which executes the code implicitly whenever a relevant task has been performed on the web server.Design:
<%@ Application Language="C#" %>
90) Enterprise Library in ASP.NET?
Enterprise Library: It is a collection of application blocks and core infrastructure. Enterprise library is the reusable software component designed for assisting the software developers.
We use the Enterprise Library when we want to build application blocks intended for the use of developers who create complex enterprise level application.
Enterprise Library Application Blocks
Security Application Block
Security Application Block provide developers to incorporate security functionality in the application. This application can use various blocks such as authenticating and authorizing users against the database.
Exception Handling Application Block
This block provides the developers to create consistency for processing the error that occur throughout the layers of Enterprise Application.
Cryptography Application Block
Cryptography application blocks provides developers to add encryption and hashing functionality in the applications.
Caching Application Block
Caching Application Block allows developers to incorporate local cache in the applications.
91)
Authentication: Prove genuineness
Authorization: process of granting approval or permission on resources.
In ASP.NET Authentication means to identify the user or in other words its nothing but to validate that he exists in your database and he is the proper user.
Authorization means does he have access to a particular resource on the IIS website. A resource can be an ASP.NET web page, media files (MP4, GIF, JPEG etc), compressed file (ZIP, RAR) etc.
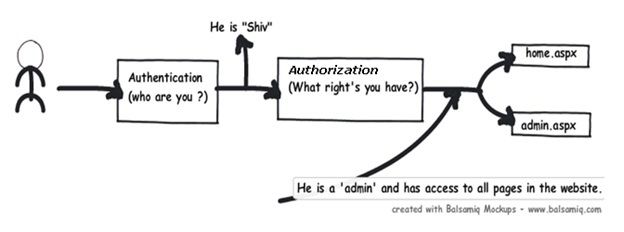
Types of authentication and authorization in ASP.NET
There are three ways of doing authentication and authorization in ASP.NET:
Windows authentication: In this methodology ASP.NET web pages will use local windows users and groups to authenticate and authorize resources.
Forms Authentication: This is a cookie based authentication where username and password are stored on client machines as cookie files or they are sent through URL for every request. Form-based authentication presents the user with an HTML-based Web page that prompts the user for credentials.
Passport authentication: Passport authentication is based on the passport website provided by the Microsoft .So when user logins with credentials it will be reached to the passport website ( i.e. hotmail,devhood,windows live etc) where authentication will happen. If Authentication is successful it will return a token to your website.
Anonymous access: If you do not want any kind of authentication then you will go for Anonymous access.
In 'web.config' file set the authentication mode to 'Windows' as shown in the below code snippets.
89) Describe application state management in ASP.NET?
Application Level State Management is used to maintain the state of all the users accessing the web forms present within the website.
The value assigned for an application is considered as an object.
Application object will not have any default expiration period.
Whenever the webserver has been restarted or stopped then the information maintained by the application object will be lost.
If any data is stored on the application object then that information will be shared upon all the users accessing the webserver.
Since the information is shared among all the users, it is advisable to lock and unlock the application object as per requirement.
Global Application Class(Global.asax):
It is a Class which consists of event handlers which executes the code implicitly whenever a relevant task has been performed on the web server.Design:
<%@ Application Language="C#" %>
90) Enterprise Library in ASP.NET?
Enterprise Library: It is a collection of application blocks and core infrastructure. Enterprise library is the reusable software component designed for assisting the software developers.
We use the Enterprise Library when we want to build application blocks intended for the use of developers who create complex enterprise level application.
Enterprise Library Application Blocks
Security Application Block
Security Application Block provide developers to incorporate security functionality in the application. This application can use various blocks such as authenticating and authorizing users against the database.
Exception Handling Application Block
This block provides the developers to create consistency for processing the error that occur throughout the layers of Enterprise Application.
Cryptography Application Block
Cryptography application blocks provides developers to add encryption and hashing functionality in the applications.
Caching Application Block
Caching Application Block allows developers to incorporate local cache in the applications.
91)
No comments:
Post a Comment